Video Lesson 03
This semester our class will be running 1x per week, online. In order to make the most of our weekly meeting, and to prevent "Zoom Fatigue", I will be teaching the class using a "flipped classroom" model. Here's how this model will operate in our class this semester:
- Before most classes you will be asked to watch a video lesson - the lesson for this week can be found below.
- After you watch all of the videos in today's lesson you will have a small task to perform - we will refer to these as "micro assignments". These "micro" assignments are fairly straightforward and short and are directly related to the content covered in the video lesson for the week.
- These "micro" assignments are due before our next class meeting. You cannot turn in a "micro" assignment late. We are setting up these assignments in this way so that everyone has a baseline level of knowledge of the topics being covered in the video lesson. This will allow us to make the most of our time together during our weekly course meeting.
- During our course meeting we will cover new topics during the first half of class. During the second half of class we will focus on working on a larger programming assignment together and in small groups.
Working with CSS classes using JavaScript
DOM queries that return multiple elements
Creating & adding elements programmatically
Micro Assignment #03
Your task is to edit the HTML document below to include some JavaScript that will make some changes to the page. Here is the code you will be working with:
<!doctype html> <html> <head> <title>Micro 03</title> <style> /* you may not make any changes to the CSS rules below */ .blue { color: blue; } .pink { color: pink; } .square { width: 100px; height: 100px; border: 1px solid black; box-sizing: border-box; display: inline-block; font-size: 200%; margin: 0px; } .yellow { background-color: yellow; } .red { background-color: red; } .orange { background-color: orange; } .container { width: 502px; height: 202px; border: 1px solid black; box-sizing: border-box; margin-top: 20px; margin-bottom: 20px; } </style> </head> <body> <!-- you may not directly change any HTML on this page you can only use JavaScript in the 'script' tag below to make changes --> <div id="box1"> <div class="square yellow">1</div> <div class="square yellow">3</div> <div class="square yellow">4</div> <div class="square yellow">2</div> <div class="square yellow">5</div> </div> <div id="box2"> <div class="square red">6</div> <div class="square red">9</div> <div class="square red">8</div> <div class="square red">10</div> <div class="square red">7</div> </div> <div id="box4" class="container"></div> <div id="box5" class="container"></div> <script> // Task #1: change all yellow squares to red square, // and all red square to yellow squares. you must use the // provided classes for this (you cannot use inline styles) // HINT: use 'querySelectorAll' instead of 'getElementsByClassName' here // this was not mentioned in the video, but 'querySelectorAll' will return // an array of elements that represents a 'snapshot' in time of all elements // that are members of a class, whereas 'getElementsByClassName' will return an // 'auto-updating' object that will change whenever the class membership // of an element changes. // Task #2: change all squares that have even numbers in them // so that they use the 'blue' class, and all odd numbered // squares so that they use the 'pink' class // Task #3: add 10 divs with classes of 'orange' and 'square' // to the div with an id of 'box4' // Task #4: add 10 divs with a class of 'square' and a RANDOMLY // selected class of orange, red or yellow to the div // with an id of box5 </script> </body> </html>
Your finished product should look like the following image:
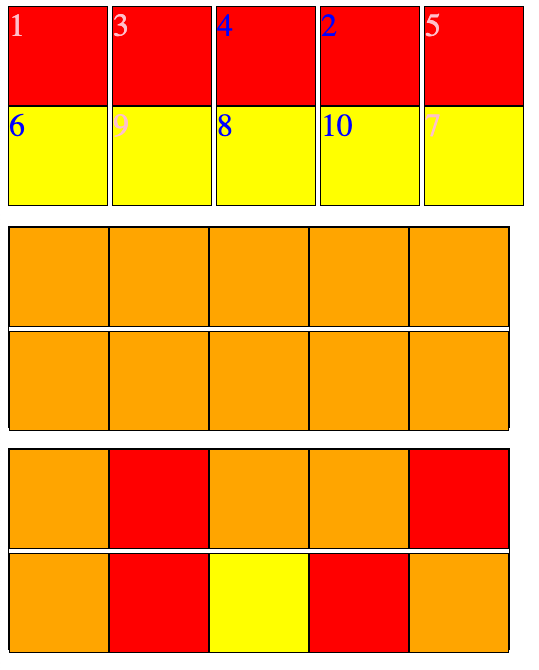
Here are some hints to get you started:
- You can ONLY make changes to the script tag - no changes to HTML, CSS or JavaScript outside of this tag is allowed.
- You will be using a number of new DOM methods that are discussed in the videos above.
- You cannot "hard-code" any values using inline styles - if the program says to add a class to an element you cannot use
element.style
to simulate this behavior.
When you are finished please upload a copy of this file to your i6 website using the process described in Assignment 01. Name your file micro_assignment_03.html
and ensure that it is accessible from within your webdev
folder. You will also want to submit a link to your work on NYU Classes under the 'Assignments' tool. You will want to include this project as a link on your homepage (also described in Assignment 01)